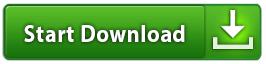
Program kalkulator menggunakan bahasa C# | Program pemula
Pembuatan program kalkulator memakai bahasa C#
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Tugas_kalkulator
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
public int jumlah(int a, int b)
{
int hasil = a + b;
return hasil;
}
public int kurang(int a, int b)
{
int hasil = a - b;
return hasil;
}
public int kali(int a, int b)
{
int hasil = a * b;
return hasil;
}
public int bagi(int a, int b)
{
int hasil = a / b;
return hasil;
}
private void button1_Click(object sender, EventArgs e)
{
if (label6.Text == "+")
{
int a, b;
a = Convert.ToInt16(label5.Text);
b = Convert.ToInt16(textBox1.Text);
textBox1.Text = Convert.ToString(jumlah(a, b));
}
if (label6.Text == "-")
{
int a, b;
a = Convert.ToInt16(label5.Text);
b = Convert.ToInt16(textBox1.Text);
textBox1.Text = Convert.ToString(kurang(a, b));
}
if (label6.Text == "*")
{
int a, b;
a = Convert.ToInt16(label5.Text);
b = Convert.ToInt16(textBox1.Text);
textBox1.Text = Convert.ToString(kali(a, b));
}
if (label6.Text == "/")
{
int a, b;
a = Convert.ToInt16(label5.Text);
b = Convert.ToInt16(textBox1.Text);
textBox1.Text = Convert.ToString(bagi(a, b));
}
}
private void button8_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = ".";
}
else
{
textBox1.Text = textBox1.Text + ".";
}
}
private void button9_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = "00";
}
else
{
textBox1.Text = textBox1.Text + "00";
}
}
private void button6_Click(object sender, EventArgs e)
{
textBox1.Text = "";
label5.Text = "";
label6.Text = "";
}
private void button10_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = "1";
}
else
{
textBox1.Text = textBox1.Text + "1";
}
}
private void button13_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = "2";
}
else
{
textBox1.Text = textBox1.Text + "2";
}
}
private void button16_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = "3";
}
else
{
textBox1.Text = textBox1.Text + "3";
}
}
private void button11_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = "4";
}
else
{
textBox1.Text = textBox1.Text + "4";
}
}
private void button14_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = "5";
}
else
{
textBox1.Text = textBox1.Text + "5";
}
}
private void button17_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = "6";
}
else
{
textBox1.Text = textBox1.Text + "6";
}
}
private void button12_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = "7";
}
else
{
textBox1.Text = textBox1.Text + "7";
}
}
private void button15_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = "8";
}
else
{
textBox1.Text = textBox1.Text + "8";
}
}
private void button18_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = "9";
}
else
{
textBox1.Text = textBox1.Text + "9";
}
}
private void button7_Click(object sender, EventArgs e)
{
if (textBox1.Text == "+")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "-")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "*")
{
label6.Text = textBox1.Text;
textBox1.Text = "";
}
if (textBox1.Text == "/")
{
label6.Text = textBox1.Text;
textBox1.Text = "0";
}
else
{
textBox1.Text = textBox1.Text + "0";
}
}
private void button2_Click(object sender, EventArgs e)
{
label5.Text = textBox1.Text;
textBox1.Text = "+";
}
private void button3_Click(object sender, EventArgs e)
{
label5.Text = textBox1.Text;
textBox1.Text = "-";
}
private void button4_Click(object sender, EventArgs e)
{
label5.Text = textBox1.Text;
textBox1.Text = "*";
}
private void button5_Click(object sender, EventArgs e)
{
label5.Text = textBox1.Text;
textBox1.Text = "/";
}
}
}
Ingin download , silahkan klik Start Download
Terus kunjungi blog pion43
http://pion43.blogspot.com
Ingin download , silahkan klik Start Download
Terus kunjungi blog pion43
http://pion43.blogspot.com
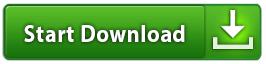
Program kalkulator menggunakan bahasa C# | Program pemula
EmoticonEmoticon